AlertDialog
showDialog(
context: context,
barrierDismissible: false, // 사용자가 다이얼로그 바깥을 터치하면 닫히지 않음
builder: (BuildContext context) {
return AlertDialog(
title: Text('제목'),
content: SingleChildScrollView(
child: ListBody(
children: <Widget>[
Text('Alert Dialog입니다'),
Text('OK를 눌러 닫습니다.'),
],
),
),
actions: <Widget>[
FlatButton(
child: Text('OK'),
onPressed: () {
// 다이얼로그 닫기
Navigator.of(context).pop();
},
),
FlatButton(
child: Text('Cancel'),
onPressed:() {
// 다이얼로그 닫기
Navigator.of(context).pop();
},
),
],
);
}
);
💬 AlertDialog 클래스는 title과 content, actions 영역을 정의해준다.
◻️ title : 제목 영역
◻️ content : 내용 영역
◻️ SingleChildScrollView와 ListBody 클래스를 사용하여 ListView와 동일한 효과를 가지도록 함
◻️ actions : 버튼 영역
DatePicker
💬 날짜를 선택할 때 사용하는 위젯
Future<DateTime?> selectedDate = showDatePicker(
context: context,
initialDate: DateTime.new(), // 초깃값 : 오늘 날짜
firstDate: DateTime(2018), // 시작일 2018년 1월 1일
lastDate: DateTime(2030), // 마지막일 2030년 1월 1일
builder: (BuildContext context, Widget child) {
return Theme(
data: ThemeData.dark(), // 다크 테마
child: child!,
);
},
);
💬 builder 프로퍼티는 테마를 설정할 때 사용한다. builder 프로퍼티를 정의하지 않으면 기본 형태로 표시된다.
💬 Future<DateTime> 타입은 미래에 DateTime 타입의 데이터를 받는다는 것을 정의한 특별한 타입이다.
◻️ Future 타입은 값이 결정될 때까지 앱이 멈추지 않으며, then() 메서드를 통해 값이 결정되었을 때의 처리를 할 수 있어서 오래 걸리는 처리를 할 때 사용한다.
예제
더보기
더보기
더보기
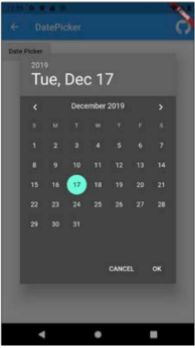
// State 클래스의 필드에 작성
DateTime? _selectedTime;
...
// Scaffold의 body에 작성
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('DatePicker'),
),
body: Column(
children: <Widget>[
ElevatedButton(
onPressed: () {
Future<DateTime?> selectedDate = showDatePicker(
context: context,
initialDate: DateTime.now(), // 초깃값
firstDate: DateTime(2018), // 시작일
lastDate: DateTime(2030), // 마지막일
builder: (BuildContext context, Widget? child) {
return Theme(
data: ThemeData.dark(), // 다크테마
child: child!,
);
},
);
// 여기서 사용자가 날짜를 선택할 때까지 코드가 블록됨
// then() 메서드를 사용하여 결과를 받는 함수를 작성한다.
selectedDate.then((dateTime) {
setState(() {
_selectedTime = dateTime!;
});
});
},
child: Text('Date Picker'),
), // ElevatedButton
Text('$_selectedTime'),
],
),
);
}
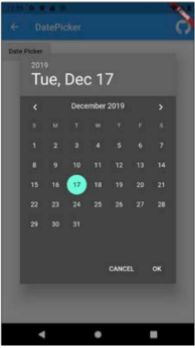
TimePicker
💬 시간을 선택할 때 사용하는 위젯
Future<TimeOfDay> selectedTime = showTimePicker(
initialTime: TimeOfDay.now();
context: context,
);
💬 initialTime 프로퍼티에 초깃값을 지정한다. TimeOfDay.now() 함수를 사용하면 현재 시간을 설정할 수 있다.
◻️ TimeOfDay 클래스에는 시간과 분 정보가 들어있다.
💬 context가 필요하며 Future 타입으로 TimeOfDay 타입의 값을 반환한다.
예제
더보기
더보기
더보기
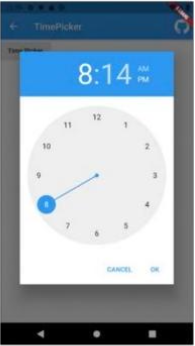
// State 클래스의 필드에 작성
String? _selectedTime;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('DatePicker'),
),
body: Column(
children: <Widget>[
ElevatedButton(
onPressed: () {
Future<TimeOfDay?> selectedTime = showTimePicker(
initialTime: TimeOfDay.now(),
context: context,
);
// 여기서 사용자가 시간을 선택할 때까지 코드가 블록됨
selectedTime.then((timeOfDay) {
setState(() {
_selectedTime = '${timeOfDay?.hour}:${timeOfDay?.minute}';
});
});
},
child: Text('Time Picker'),
),
Text('$_selectedTime'),
],
),
);
}
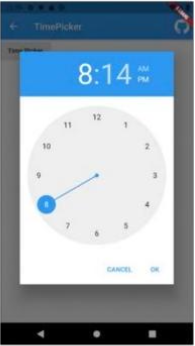
'Flutter > Concept' 카테고리의 다른 글
[ Flutter ] 쿠퍼티노 디자인 (0) | 2021.12.29 |
---|---|
[ Flutter ] 애니메이션 (0) | 2021.12.29 |
[ Flutter ] 버튼 (0) | 2021.12.28 |
[ Flutter ] 화면 배치 (0) | 2021.12.28 |
[ Flutter ] 초기 설정 (0) | 2021.12.20 |